SPRING, a powerful framework!
Web development has become an essential part of modern business, and building a high-quality web application requires a powerful framework. Spring is one such framework that offers a wide range of features to simplify web application development, from dependency injection and aspect-oriented programming to security and transaction management.

What is Spring?
- It is a framework that provides a programming and configuration model for java based applications.
- It focuses on “Plumbing”: which refers to the infrastructure, that connects and manages the different components and services in a software application. The goal of this “plumbing” is to make it easier to develop, maintain, and test the application.
- It is a lightweight framework that addresses each tier in a web app:
-Presentation Layer: MVC
-Buisness Layer: IoC container and AOP support.
-Persistence Layer: DOA template support for ORMs and JDBC.
- It is Complementary in managing implementations of sub-systems through its core distribution of classes and a configuration file:
-RestServices: create services.
-Persistence: create template classes to talk to the database (database connection)
-Security: Java Security
Why Spring?
- It makes programming Java quicker, easier, and safer for everybody with SPRING SECURITY.
- It focuses on speed, simplicity, and productivity.
- It is the world’s most popular Java framework.
Dependency Injection?
- Dependency injection is a pattern used to create instances of objects that have delegation patterns to other objects.
- Can be achieved via setter and/or constructor injection technique and/or annotation (Autowiring).
- It is a simple mechanism to implement within the framework of object-oriented programming and which makes it possible to decrease the coupling between two or more objects.
- It implements IoC by allowing objects to receive their dependencies from external sources, instead of hard-coding them within the class. This leads to a loosely-coupled design, where the dependencies can be easily changed or swapped out without affecting the rest of the code.
XML-based or Java-based configuration in Spring?
It ultimately depends on the needs and preferences of the team and the project you are working on.
Here are some pros and cons of each configuration approach:
1.XML-based Configuration:
Pros:
- Familiarity: XML is widely used and well-known to most developers.
- Tool Support: There are many tools available that make it easier to work with XML configuration.
- Separation of Concerns: XML configuration can be kept separate from Java code, which can make it easier to maintain.
Cons:
- Verbosity: XML files can become quite verbose, especially for complex projects.
- Readability: XML files can be difficult to read and understand for developers who are not familiar with the format.
2. Java-based Configuration:
Pros:
- Conciseness: Java code is generally more concise than XML configuration.
- Type Safety: Java configuration provides type safety, which can help catch errors early in the development process.
- Flexibility: Java code can be more flexible than XML configuration, as you have full control over the code and can take advantage of Java features like inheritance and polymorphism.
Cons:
- Steep Learning Curve: Java-based configuration may require a deeper understanding of the framework, especially for developers who are not familiar with Java.
- Poor Tool Support: There are not as many tools available to help with Java configuration as there are for XML configuration.

Spring MVC?
MVC stands for Model-View-Controller, which is a design pattern used in software engineering to separate the concerns of an application into three distinct components:
- Model: This represents the data and the business logic of the application. It is responsible for managing the data, as well as performing operations on the data such as validation, calculation, and storage.
- View: This is the visual representation of the data, responsible for presenting the data to the user. It is typically implemented as a user interface, such as a web page or a graphical user interface.
- Controller: This acts as an intermediary between the Model and the View, receiving user input from the View and using it to update the Model. It is responsible for interpreting user actions, such as button clicks, and updating the Model accordingly.
The purpose of the MVC design pattern is to promote the separation of concerns and modularity in the code. This makes it easier to maintain and extend the application over time, as changes to one component can be made independently of the other components. Additionally, it also makes it easier to test individual components, as they can be tested in isolation from the other components.
In Spring MVC, the DispatcherServlet acts as the front controller and is responsible for coordinating the flow of control between different components in the application, such as controllers, view resolvers, and model objects. The DispatcherServlet is typically defined in the web.xml file and is responsible for processing incoming HTTP requests and mapping them to the appropriate controller for handling.
How does the DispatcherServlet locate Controllers?
The DispatcherServlet, which is the front controller in a Spring MVC application, locates controllers using the Handler Mapping.
The HandlerMapping is responsible for mapping incoming requests to appropriate handlers, such as controllers, that can handle the request and produce a response. When a request is received by the DispatcherServlet, it uses the Handler Mapping to determine which controller should handle the request.
The DispatcherServlet obtains the HandlerMapping from the application context. In a Spring Boot application, the framework automatically configures a RequestMappingHandlerMapping, which uses the @RequestMapping annotation on the controller to map requests to handlers.
Once the DispatcherServlet has determined the appropriate controller, it uses the controller to handle the request and produce a response. The response is then returned to the client through the DispatcherServlet.
Spring and JPA
JPA (Java Persistence API) is a specification for object-relational mapping (ORM) in Java.
We can use JDBC, java database connectivity with JC template or we can use complete or object-relational mapping technologies ORM such as Hibernate.
Hibernate is an open-source persistence framework tool for relational database objects.
• It manages the Mapping between the objects of the application and the database data.
• The use of JPA / HIBERNATE allows us to save developing time:
– Automatic generation of SQL code (CRUD).
– Automatic generation of tables from Java entities.
Spring AOP
Aspect-Oriented Programming, allows you to separate cross-cutting concerns (such as logging, security, and transaction management) from the core business logic of an application.
Spring AOP can be used for a variety of cross-cutting concerns, such as logging, caching, transaction management, security, and performance monitoring. By separating these concerns from the core business logic of an application, Spring AOP helps to improve code modularity, maintainability, and reusability.
Transactions
Using transactions in Spring Boot can greatly simplify the process of managing database operations, ensuring data consistency and integrity, and reducing the likelihood of errors and data corruption.
You can use the @Transactional annotation. When a method annotated with @Transactional is called, Spring will automatically create a new transaction, and all database operations performed within the method will be part of the transaction.
Spring Cloud Overview
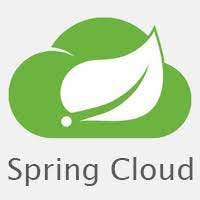
MicroServices
Monolithic applications can be successful!
However, when a change is made to just a small part of the application, you have to redeploy and rebuild the entire monolith only because it’s a single entity.
A microservice architecture style is an alternative where applications are built via a suite of services that communicate with each other.
The aim is that the services are independent of deployment, replaceable, upgradeable, and scalable. Here we could scale one service up because that’s where all the traffic is going and not have to scale all the other services up to the same frequency.
With microservices, the consequences, service being decoupled, independently deployable, and replaceable, we can scale our services at different frequencies across single or multiple services.
This helps out with resources because a specific service that we know takes up a lot of traffic. We would scale it at a far greater rate than one that receives very little traffic. Not only that, the service has become reusable in different workflows.

Service Registry
Eureka is a service discovery tool developed by Netflix. It is an open-source component of Netflix’s cloud platform, and it is designed to help manage service instances and provide a way for services to find each other.
With Eureka, each service registers itself with the Eureka server when it starts up, and the Eureka server maintains a dynamic directory of all available services and their instances. This allows services to discover and communicate with each other without needing to know the physical location or IP address of other services.
Eureka provides several features to help manage and monitor the services in the system, including:
- Service registration and discovery: Services can register themselves with Eureka and discover other services in the system.
- Load balancing: Eureka can be used to perform client-side load balancing, allowing clients to distribute requests across multiple instances of a service.
- Health checking: Eureka provides health checks to ensure that services are available and functional, and can remove instances that are not responding.
- Metrics: Eureka provides metrics and monitoring capabilities to help identify and diagnose issues in the system.
Circuit Breaker
Circuit Breaker is a design pattern used to improve the resilience and fault-tolerance of distributed systems. The Circuit Breaker pattern is implemented using the Hystrix library, which provides an implementation of the pattern for Spring applications.
In a nutshell, the Circuit Breaker pattern monitors the calls made to a service and breaks the circuit (i.e., stops the calls) when the service is not responding or is responding with errors. This prevents the service from being overloaded and potentially crashing and allows the system to gracefully degrade when a service is unavailable.
When the Circuit Breaker is tripped, Hystrix can provide a fallback response or use a cached response, depending on the configuration. Once the service is deemed healthy again, the Circuit Breaker can be reset and calls to the service can resume.
Using the Circuit Breaker pattern in Spring applications can improve the overall reliability and availability of the system, especially in the face of network failures or high loads.
Feign
Feign, is a declarative REST client that simplifies the process of invoking RESTful APIs. It allows you to declare the HTTP API you want to consume as an interface, and Spring Cloud Feign will automatically generate an implementation of that interface that uses Ribbon for load balancing and Hystrix for fault tolerance. This makes it easy to write client-side code that can consume RESTful APIs without having to worry about the details of how the HTTP requests are made.
Zuul
Zuul is a proxy server that acts as a gateway to your microservices-based architecture. It provides dynamic routing, monitoring, resiliency, and security capabilities to your applications. With Zuul, you can easily route requests to your microservices, implement load balancing, and protect your microservices by providing authentication and authorization.
In a Spring Cloud-based application, you can use Zuul and Feign together to create a powerful and scalable microservices architecture. Zuul can act as a gateway to your microservices, and Feign can be used to consume those services in a declarative way. Additionally, both Zuul and Feign integrate with Eureka (the Netflix Service Registry) and Ribbon (the Netflix Client-Side Load Balancer), making it easy to create a fully functional microservices architecture.
Spring is a powerful and versatile framework that simplifies Java application development. Its wide range of functionalities makes it easy to build robust, scalable, and efficient applications that meet the needs of modern businesses. From dependency injection to aspect-oriented programming, Spring provides developers with the tools they need to focus on business logic while the framework takes care of low-level details. Whether you’re building a small application or a large-scale enterprise system, Spring can help you achieve your goals quickly and efficiently. So, if you haven’t already, it’s time to start exploring the many functionalities of Spring and take your Java development to the next level.